Simple Python Guide For Beginners
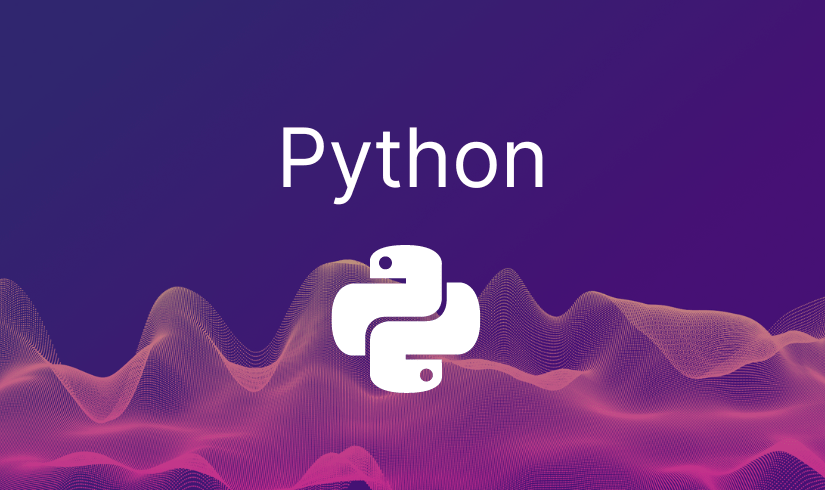
Python Basics and Syntax
Introduction
In this section, you will learn the basics of Python programming and its syntax. We will cover fundamental concepts such as comments and documentation, indentation, print statement, input from users, and basic operators.
Comments and Documentation
In Python, comments are used to add explanatory notes to the code and improve its readability. They are ignored by the interpreter when the code is executed. Here’s an example:
# This is a single-line comment """ This is a multi-line comment. It can span multiple lines. """ # Documenting a function def add(a, b): """ This function adds two numbers. Parameters: a (int): The first number. b (int): The second number. Returns: int: The sum of the two numbers. """ return a + b
Comments are helpful for understanding code and providing additional information about functions, classes, or modules. They improve code maintainability and facilitate collaboration.