Simple Python Guide For Beginners
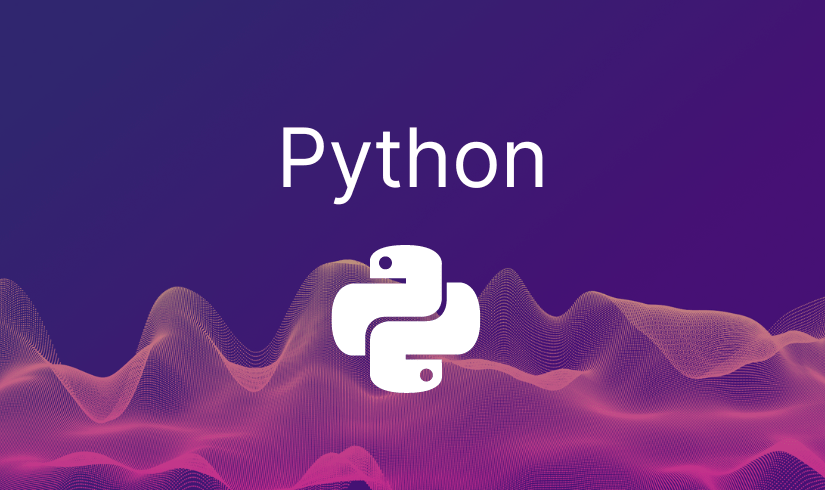
Working with Sets
In this section, you will learn about sets in Python. Sets are unordered collections of unique elements. We will cover topics such as creating sets, adding and removing elements, set operations, set methods, and practical use cases for sets.
Creating Sets
In Python, you can create sets by enclosing elements in curly braces {} or by using the `set()` function. Here are some examples:
fruits = {"apple", "banana", "cherry"} numbers = set([1, 2, 3, 4, 5]) mixed = set([1, "apple", True])
Adding and Removing Elements
You can add elements to a set using the `add()` method, and remove elements using the `remove()` or `discard()` methods. Here’s an example:
fruits = {"apple", "banana", "cherry"} fruits.add("orange") # Add element fruits.remove("banana") # Remove element print(fruits) # Output: {'apple', 'cherry', 'orange'}
Set Operations
Python provides several operations that can be performed on sets. These include union, intersection, difference, and symmetric difference. Here are some examples:
set1 = {1, 2, 3, 4} set2 = {3, 4, 5, 6} union = set1.union(set2) # Union intersection = set1.intersection(set2) # Intersection difference = set1.difference(set2) # Difference (set1 - set2) symmetric_difference = set1.symmetric_difference(set2) # Symmetric Difference print(union) # Output: {1, 2, 3, 4, 5, 6} print(intersection) # Output: {3, 4} print(difference) # Output: {1, 2} print(symmetric_difference) # Output: {1, 2, 5, 6}
Set Methods
Python provides several built-in methods that can be used with sets. Here are a few commonly used set methods:
fruits = {"apple", "banana", "cherry"} fruits.add("orange") # Add an element fruits.remove("banana") # Remove an element fruits.clear() # Remove all elements length = len(fruits) # Get the length of the set is_present = "apple" in fruits # Check if an element is present print(fruits) # Output: set() print(length) # Output: 0 print(is_present) # Output: False
Practical Use Cases for Sets
Sets are useful for various purposes such as removing duplicates from a list, checking for membership, performing mathematical operations, and more. Here are some practical use cases for sets:
fruits = ["apple", "banana", "cherry", "apple", "banana"] unique_fruits = set(fruits) is_present = "apple" in unique_fruits print(unique_fruits) # Output: {'banana', 'apple', 'cherry'} print(is_present) # Output: True