Simple Python Guide For Beginners
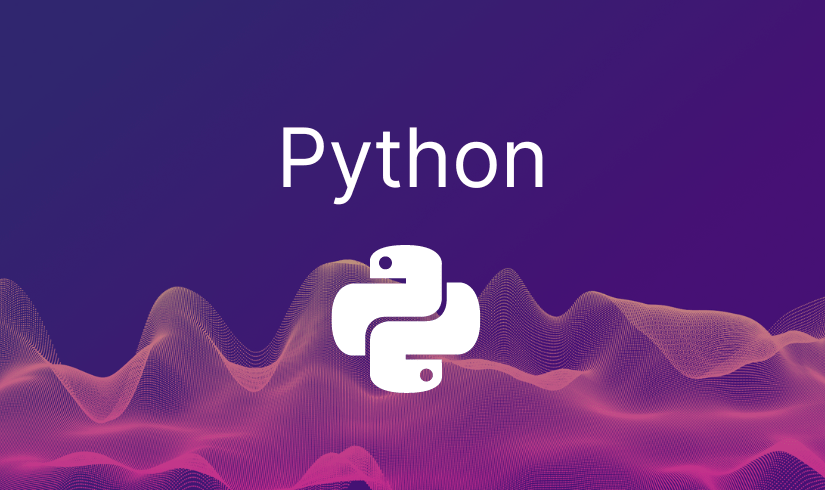
File Handling
In this section, you will learn about file handling in Python. File handling allows you to read from and write to files on your computer. We will cover topics such as opening and closing files, reading from and writing to files, file modes, and common file operations.
Opening and Closing Files
You can open a file in Python using the `open()` function, which takes the file name and the mode as parameters. Here’s an example:
file = open("example.txt", "r") # Perform file operations... file.close()
Reading from Files
You can read the contents of a file using various methods. The most common method is the `read()` method, which reads the entire file. Here’s an example:
file = open("example.txt", "r") content = file.read() print(content) file.close()
Writing to Files
You can write to a file using the `write()` method. If the file does not exist, it will be created. If it already exists, its contents will be overwritten. Here’s an example:
file = open("example.txt", "w") file.write("Hello, world!") file.close()
File Modes
When opening a file, you can specify different modes to control the file’s behavior. Here are some commonly used file modes:
file = open("example.txt", "r") # Read mode file = open("example.txt", "w") # Write mode (overwrite existing content) file = open("example.txt", "a") # Append mode (append to existing content) file = open("example.txt", "x") # Exclusive creation mode (fails if the file already exists)
Common File Operations
Here are some common file operations you can perform:
file = open("example.txt", "r") # Read line by line line1 = file.readline() line2 = file.readline() # Iterate over lines for line in file: print(line) # Close the file file.close()