Simple Python Guide For Beginners
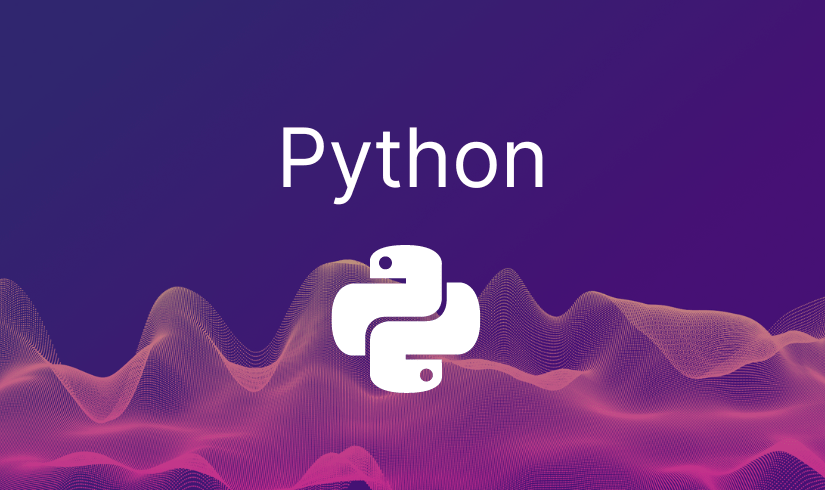
Numpy Library
Creating Numpy Arrays
Numpy is a powerful Python library for numerical computing. It provides support for efficient array operations and mathematical functions. Numpy is widely used in scientific computing, data analysis, and machine learning. In this section, we will explore some of the key features and functionalities of the Numpy library.
Numpy arrays are used to store and manipulate homogeneous data efficiently. You can create Numpy arrays using various methods, such as converting lists or tuples to arrays, using built-in functions, or reading data from files.
import numpy as np # Create an array from a list my_list = [1, 2, 3, 4, 5] my_array = np.array(my_list) # Create an array of zeros zeros_array = np.zeros(5) # Create an array of ones ones_array = np.ones(5) # Create a range of values range_array = np.arange(1, 10, 2) # Read data from a file data_array = np.loadtxt('data.txt')
Array Operations
Numpy provides a wide range of array operations for performing mathematical calculations and manipulations on arrays. You can perform element-wise operations, array broadcasting, array reshaping, and more.import numpy as np# Perform element-wise operationsa = np.array([1, 2, 3])b = np.array([4, 5, 6])c = a + b# Perform array broadcastingd = np.array([[1, 2, 3], [4, 5, 6]])e = np.array([1, 2, 3])f = d + e# Reshape an arrayg = np.array([1, 2, 3, 4, 5, 6])h = g.reshape(2, 3)
Mathematical Functions
Numpy provides a wide range of mathematical functions for performing calculations on arrays. These functions can be applied to individual elements or entire arrays.
import numpy as np # Apply mathematical functions a = np.array([1, 2, 3, 4, 5]) mean = np.mean(a)median = np.median(a) std_dev = np.std(a) max_value = np.max(a) min_value = np.min(a)