Simple Python Guide For Beginners
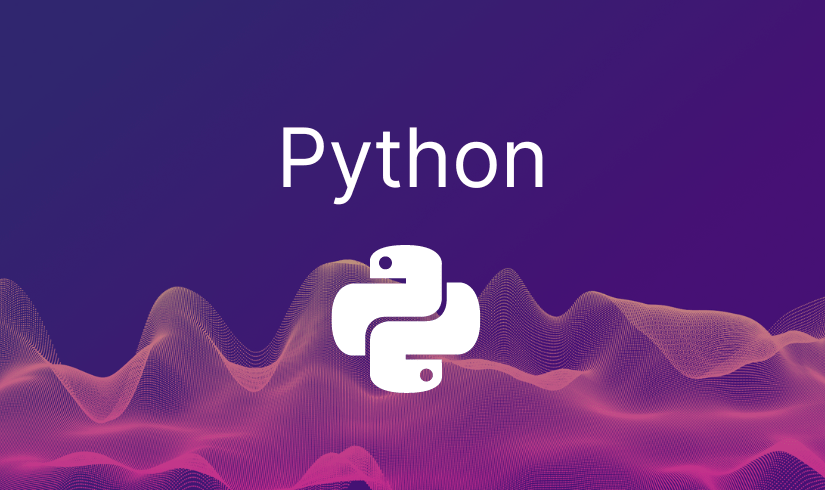
Variables and Data Types
In this section, you will learn about variables and data types in Python. Variables are used to store and manipulate data, while data types define the nature of the data being stored. We will cover topics such as variable declaration and assignment, numeric data types, strings, Boolean data type, and type conversion.
Variable Declaration and Assignment
name = "John" age = 25
Numeric Data Types
x = 10 y = 3.14 z = 2 + 3j
Strings
message = "Hello, World!"
Boolean Data Type
is_true = True
Type Conversion
a = 10 b = float(a) c = str(a) d = bool(a)
Python Tokens
In Python, a token is the smallest individual unit of a program. It could be a keyword, an identifier, a literal, a punctuation symbol, or an operator. Understanding Python tokens is essential for writing and understanding Python code. In this section, we will explore different types of Python tokens.
Keywords
Keywords are reserved words in Python that have a predefined meaning and cannot be used as identifiers (variable names or function names). Examples of keywords include `if`, `else`, `for`, `while`, `def`, `class`, and many more.
if x > 5: print("x is greater than 5") else: print("x is not greater than 5")
Identifiers
Identifiers are names given to entities such as variables, functions, classes, modules, etc. In Python, an identifier must follow certain rules, such as starting with a letter or underscore, and can contain letters, digits, or underscores. It is case-sensitive.
x = 10 name = "Alice" def greet(): print("Hello, " + name)
Literals
Literals are constant values assigned to variables or used directly in the code. They represent fixed values such as numbers, strings, and boolean values.
x = 10 # Integer literal name = "Alice" # String literal is_active = True # Boolean literal
Punctuation Symbols
Punctuation symbols are special characters used for syntax and structure in Python code. They include parentheses, commas, colons, semicolons, brackets, etc.
x = (1, 2, 3) # Parentheses name = "Alice" # Quotation marks
Operators
Operators are symbols used to perform operations on operands. Python supports various types of operators, such as arithmetic operators, comparison operators, logical operators, assignment operators, etc.
x = 10 + 5 # Addition operator y = x > 5 # Comparison operator is_valid = x > 0 and y # Logical operator