Simple Python Guide For Beginners
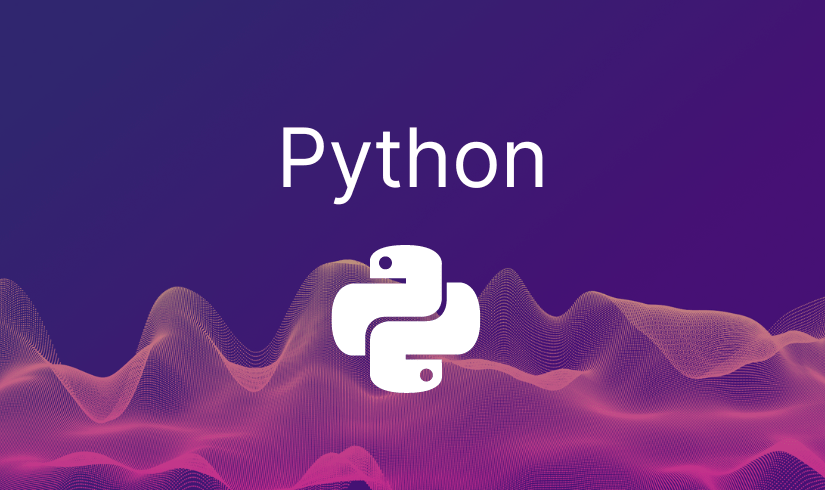
Loops
In this section, you will learn about loops in Python. Loops are used to iterate over a sequence of elements or perform a set of instructions repeatedly. We will cover topics such as the `for` loop, the `while` loop, loop control statements (such as `break` and `continue`), and nested loops.
The `for` Loop
fruits = ["apple", "banana", "cherry"] for fruit in fruits: print(fruit)
The `while` Loop
count = 0 while count < 5: print(count) count += 1
Loop Control Statements
for number in range(10): if number == 3: continue if number == 8: break print(number)
Nested Loops
for x in range(1, 4): for y in range(1, 3): print(x, y)