Simple Python Guide For Beginners
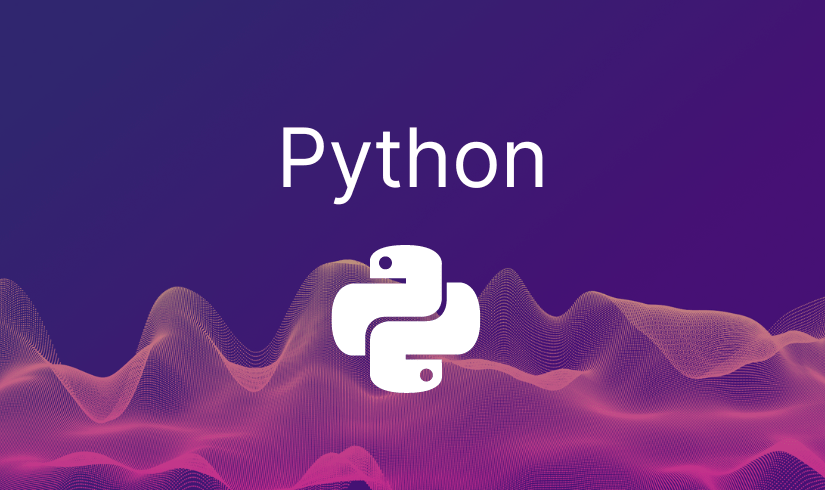
Strings
In this section, you will learn about strings in Python. Strings are sequences of characters, and they are used to represent textual data. We will cover topics such as creating strings, accessing characters in a string, string concatenation, string methods, and string formatting.
Creating Strings
In Python, you can create strings by enclosing characters in single quotes (”) or double quotes (“”). Here are some examples:
string1 = 'Hello, world!' string2 = "Python is awesome" string3 = '''This is a multi-line string'''
Accessing Characters in a String
You can access individual characters in a string using indexing. Python uses zero-based indexing, where the first character is at index 0. Here’s an example:
string = "Hello, world!" print(string[0]) # Output: H print(string[7]) # Output: w
String Concatenation
You can concatenate strings using the `+` operator. This allows you to combine multiple strings into a single string. Here’s an example:
string1 = "Hello" string2 = "world!" result = string1 + " " + string2 print(result) # Output: Hello world!
String Methods
Python provides many built-in methods to manipulate strings. Here are a few commonly used string methods:
string = "Hello, world!" print(string.upper()) # Output: HELLO, WORLD! print(string.lower()) # Output: hello, world! print(string.startswith("H")) # Output: True print(string.endswith("ld!")) # Output: True print(string.replace("o", "X")) # Output: HellX, wXrld!
String Formatting
String formatting allows you to create dynamic strings by inserting values into placeholders within a string. Here are a few examples of string formatting:
name = "Alice" age = 25 greeting = "My name is {} and I'm {} years old.".format(name, age) greeting2 = f"My name is {name} and I'm {age} years old." print(greeting) # Output: My name is Alice and I'm 25 years old. print(greeting2) # Output: My name is Alice and I'm 25 years old.